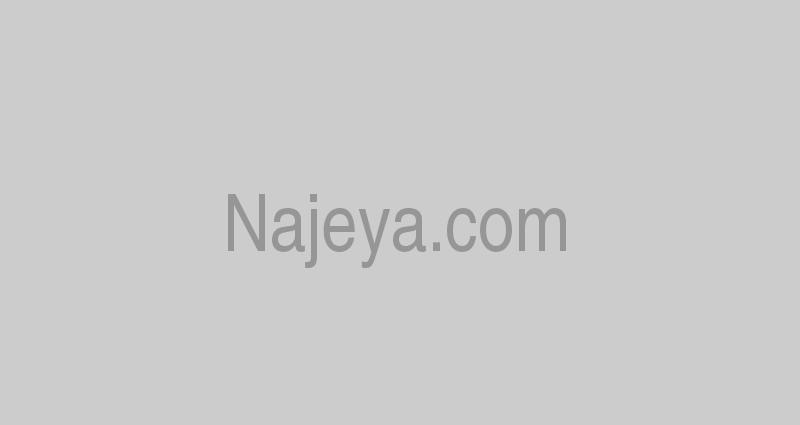
how to insert a string into another string c++
C program to insert substring into a string | Programming ... In C programming, a string is a sequence of characters terminated with a null character \0.For example: char c[] = "c string"; When the compiler encounters a sequence of characters enclosed in the double quotation marks, it appends a null character \0 at the end by default.. Memory Diagram 01-02-2011 #8. template <class InputIterator> void insert (InputIterator first, InputIterator last); It accepts a range of input iterators and one by one all the elements in set while traversing through the range( first to last-1). After getting the substring index, we split the original string and then concatenate the split strings and the string we need to insert using the . StringBuffer resString = new StringBuffer (str); resString.insert (index + 1, newSub); Let us now see an example to insert a string into another −. This method inserts the string inside another string and returns a new modified string as the result. C++ String Copy | How to Copy String in C++ with Examples The find() method will then check if the given string lies in our string. For example, the following code inserts "bbb" after the second character in str1 and the result string is "pbbbpp". Declare another variable to store copy of first string in text2. Here's a simple example: char *a = "Dany S."; char *b = "My name is *a , I come from . We can also insert a string at a specified positon in another string. In C++, we have the inbuilt data type string. C# Insert String Examples - Dot Net Perls Returns: String - The result string. The strcat function is used to concatenate one string (source) at the end of another string (destination). We will explore each method is depth but the easiest approach is to use + operator and the format() method in C++20. 2) To copy one string into another string. sublen : It defines the number of characters of string str to be inserted in another string object. The keyword INSERT INTO is used for inserting records in a table. std::set provides an another overloaded version of insert() i.e. Step 2: We append a second word to the string. newStr = insertAfter(str,pat,newText) inserts newText into str after the substring specified by pat and returns the result as newStr.If pat occurs multiple times in str, then insertAfter inserts text after every occurrence of pat.. 4.4 Strings - Racket How to add to string? - Scripting Support - DevForum | Roblox C Program to copy string using. Insert one string into another. char *ptr = strtok (str, delim); C program to copy one string to another string - Codeforwin Modifying individual characters. A protip by dfeddad about php, string, insert, and substr_replace. This enables you to do things like insert a word at a specific position. Let us see an example, Let us see the possible ways to solve the problem, By Traversing the String subpos : It defines the position of first character in string str which is to be inserted in another string object. Below is a program on strcpy () function. Input string from user and store it to some variable say text1. I'm an alien from another world. Let's see the simple example. JavaScript String slice () method: This method gets parts of a string and returns the extracted parts in a new string. If the string can be transformed, find the minimum number of operations required for the transformation. python libraries . To concatenate two strings str1 and str2, you will copy all characters of str2 at the end of str1. Parameter and Return Type The split() function exists in many programming languages to divide the string into multiple parts. Explanation: In char *a = aa;, a is a pointer to a char array and stores the base address of the aa. C program to insert a substring into a string: This code inserts a string into the source string. n : It determines the number of characters to be inserted. For example, say we have two strings: "C programming" and "language". This method places one string into another. The following example shows how to replace a set of characters in a string. (in this example, 0 because it's the beginning of the string). Suppose that we would like to add a string variable called gender. strcpy () Function. E.g. Extends the string by appending additional characters at the end of its current value: (1) string Appends a copy of str. (2) substring Appends a copy of a substring of str.The substring is the portion of str that begins at the character position subpos and spans sublen characters (or until the end of str, if either str is too short or if sublen is string::npos). Below is the step by step descriptive logic to concatenate two string. The letter is then looked at to figure out what field is supposed to go there. char delim [] = " " ; strtok accepts two strings - the first one is the string to split, the second one is a string containing all delimiters. This method belongs to the C++ string class (std::string). Example 2: Adding a string variable to an existing data set . learn 4 Methods to Insert a Variable into a String Method #1: using String concatenation Method #2: using the % operator Method #3: using the format() function Method #4: using f-string Python . String newSub = "no "; Now, the index where the new sub string will get inserted −. Sometimes we need to split the string data for programming purposes. The String class provides several ways to add, insert, and merge strings including + operator, String.Concate (), String.Join (), String.Format (), StringBuilder.Append (), and String Interpolation. Declare another variable to store copy of first string in text2. There are 2 ways to insert records in a table. 4.4 Strings. Method 1: A string is a fixed-length array of characters.. A string can be mutable or immutable.When an immutable string is provided to a procedure like string-set!, the exn:fail:contract exception is raised. C++ Strings Original handout written by Neal Kanodia and Steve Jacobson. Find length of str1 and store in some variable say i = length . Thanks for the idea. Example of character: 'a' or 'A.' C Program to insert a Sub-String in the given String at specified Position. Inserts additional characters into the string right before the character indicated by pos (or p): (1) string Inserts a copy of str. First, we need to create the new variable using the string command. It then calls the Insert method to insert the text entered by the user into a string. Concatenation involves appending one string to the end of another string. String copy is a function in C++ which is used for copying one entire string to another string seamlessly without making many changes and efforts. Returns *this. Errors: Throws out_of_range if idx > size(). In this article, We've discussed about inserting a string into another string using common approcach, stirng substring method and StringBuffer.insert() method. A string is a variable that stores a sequence of letters or other characters, such as "Hello" or "May 10th is my birthday!". strncat() is a predefined function used for string handling. Use .. at the end of a string followed by the string you want to append. Exceptions: System.ArgumentOutOfRangeException: startIndex is negative or greater than the length of . It copies string pointed to by source into the destination. This function accepts two arguments of type pointer to char or array of characters and returns a pointer to the first string i.e destination. ← Prev. C++ Program to Copy One String into Another String. Add A Character To A String In C++. The Insert method will add that content whenever you want it. C program to concatenate two strings; for example, if the two input strings are "C programming" and " language" (note the space before language), then the output will be "C programming language." To concatenate the strings, we use the strcat function of "string.h", to dot it without using the library function, see another program below. Syntax: char *strncat(char *dest, const char *src, size_t n) Parameters: This method accepts the following parameters: dest: the string where we want to append. Get the string str and character ch; Use the strncat() function to append the character ch at the end of str. And therefore, we must include the header file <string>, We must invoke this on a string object, using another string as an argument. In this program we will copy one string into another, using the strcpy () method defined under the string.h library. public String addChar(String str, char ch, int position) { StringBuilder sb = new StringBuilder (str); sb.insert (position, ch); return sb.toString (); } The above code needs to create only a single StringBuilder object to insert the character at the position. Below is the step by step descriptive logic to copy one string to another string. Here we need to concatenate str2 to str1. My question was deleted because of duplicate but this is not the case. But I didn't think of having a temporary single vector<string> to use for all my vector<string> objects I insert into the map. Just like the other data types, to create a string we Logic to copy one string to another string. There are multiple ways to add two or more strings or a string and an integer in C++. In order to use these string functions you must include string.h file in your C program. We can perform such operations using the pre-defined functions of "string.h" header file. The answer SO does not and where SO is referring to is way to complicated to implement in my solution. Another method to insert a variable into a string is using the format() function. c : Character value to insert. Add String to Array With the List.Add() Method in C. Unfortunately, there is no built-in method for adding new values to an array in C#. C# Insert String ExamplesUse the Insert method to add a substring to an existing string at an index. My example uses an already made sql-string. string copy in C++ is part of the standard library function which makes the content of one string, in fact, the entire string copied into another string. The Insert() function in String Class will insert a String in a specified index in the String instance. You can produce a character array from a string, modify the contents of the array, and then create a new string from the modified contents of the array. Inserting an Iterator Range into a Set. "; So, in string b in place of *a I exp. String constants generated by the default reader (see Reading Strings) are immutable, and they are interned in read-syntax mode. I need to insert a string in another string at a specific place. Inserting an Iterator Range into a Set. Get the Strings and the index. Obtain a substring. using namespace System; int main () { String^ animal1 = "fox"; String^ animal2 = "dog"; String^ strTarget = String::Format ( "The {0} jumps over the {1 . Example 1. Example 1. subpos : It defines the position of first character in string str which is to be inserted in another string object. All string facilities use this data type to represent positions and lengths when dealing with . This is the easiest way to insert records into a table but I strongly avoid it because it is less secure than . C did not have them as such the data type string, because of which we had to form a character array to form a string. First, it uses the String.ToCharArray () method to create an array of characters. Hey, so you probably came here wondering the same question: How to Add a character to a string in C++. As we learned in our earlier, the index method starts with zero. Logic to copy one string to another string. strcpy (destination, source) is a system defined method used to copy the source string into the destination variable. string& string::insert (size_ type idx, const char* cstr) idx : is the index number where insertion is to be made. Input The first line contains a number N (1 ≤ N ≤ 50) the number of test cases. The string with the %x symbols in it are parsed to find each symbol. Insert using Simple Query; Insert using Parameterized Query; Direct Insert Record in a Table. Programmer_P. Given two strings, determine if the first string can be transformed into the second string. Notice that source is preceded by the const modifier because strcpy () function is not allowed . Appending one string at the end of another - strcat. So let's go ahead and take a look at it. The List data structure should be used for dynamic allocation and de-allocation of values in C#. Compare two strings. Run a loop from 0 to end of string. string gender (A6). Must know - Program to find length of string. Notice that the subscripts for the individual characters of a string start at zero, and go from 0 to length−1.. Notice also the data type for the subscript, string::size_type; it is recommended that you always use this data type, provided by the string class, and adapted to the particular platform. Strings in C# and .NET Core are immutable. Given 2 string, the task is to insert one string in another at a specified position using javascript, we're going to discuss few techniques. It does the following: Takes the destination string. Working with string data is an essential part of any programming language. We can use concatenation to generate the output, "C programming language." There are a few ways we can append or concatenate strings in C. In other words, the new string should be ( S0 + T0 + S1 + T1 + .. ). There are 2 ways of solving this: View Profile View Forum Posts . Planet Earth is only my vacation home, and I'm not liking it. print ("Hello" .. "World") 3 Likes. Strings (Unicode) in The Racket Guide introduces strings. Note: If the length of S is greater than the length of T then you have to add the rest of S letters at the end of the new string and vice versa. In char *b = bb;, b is a pointer to a char array and stores the base address of the bb. Apologies for me not being clear or for me not understanding, I'm looking for a way to repeat the addition of strings such as values. *cstr : is the pointer to the C-string which is to be inserted. Syntax of String find() in C++. It allocates the same amount of memory that the original String has, but to create a . C# String Insert () The C# Insert () method is used to insert the specified string at specified index number. Input two string from user. The word "Perls" is appended to the string with the += operator. There are two way to copy one sting into another string in C++ programming language, first using pre-defined library function strcpy() and other is without using any library function. Input string from user and store it to some variable say text1. execute. We use Insert() to place one substring in the middle of a string—or at any other position. C++ Strings One of the most useful data types supplied in the C++ libraries is the string. Add/Append String to StringBuilder Use the Append() method to append a string at the end of the current StringBuilder object. Then we will assign values to the variable. #7) Insert( ) The Insert method in C# is used for inserting a string at a specific index point. [crayon-5f81359083b14657807040/] Output : [crayon-5f81359083b1e227680988/] Explanation : [crayon-5f81359083b22120489902/] Scan Entered String From Left to Right , Character by Character. std::set provides an another overloaded version of insert() i.e. Sample Solution: C Code: We first use the string.find () method to get the substring index in the string, after which we need to insert another string. The code below only inserts the first 'c' char into the place of an 'o', so I'm wondering how can I insert the entire "cc" string into the space of 'o'? In SQL Server, you can use the T-SQL STUFF () function to insert a string into another string. First few methods to know. Below is the step by step descriptive logic to copy one string to another string. In this case there is only one delimiter. sublen : It defines the number of characters of string str to be inserted in another string object. (2) substring Inserts a copy of a substring of str.The substring is the portion of str that begins at the character position subpos and spans sublen characters (or until the end of str, if either str is too short or if sublen is npos). Here, we will see how to insert to a string python.. To insert into a string we will use indexing in python. C Programming: Copy one string into another string Last update on February 26 2020 08:07:26 (UTC/GMT +8 hours) C String: Exercise-8 with Solution. sublen : It defines the number of characters of string str to be inserted in another string object. Start and end parameters are used to specify the part of . string.h is the header file required for string functions. Here's the official syntax: STUFF ( character_expression , start , length , replaceWith_expression ) character . As soon as Source or Original String Ends , Process of Coping . yumc (yumc) December 31, 2019, 4:58am #3. The AppendLine() method append a string with the newline character at the end. Must know - Program to find length of string. The code is simple and is really easy to execute so understanding it wouldn't be any problem for you at all. After inserting the specified string, it returns a new modified string. Consider the user enters two strings and an index value, we need to insert the second string at the given index of the first string. Modifying strings is an important programming skill. c : Character value to insert. Create a new String. EX: Value = (Value + 1) We will see how to compare two strings, concatenate strings, copy one string to another & perform various string manipulation operations. So the first time it is called, it will point to the first word. String Declaration. Step 1: A string reference is assigned the literal value "Dot Net ". But, if we have a filled array containing some important data and want to add a new element to the array, we can . Run a loop from 0 to end of string. Then the string is changed by removing the %x and whatever field is requested replaces it. Remove. Then insert the string to be inserted into the string. strtok returns a pointer to the character of next token. a) Iterate the for loop with the structure for(i=0;s[i]!='\0′;i++) copy the elements of the string s1 into the string s2 as s2[i]=s1[i] until the last element of string s1. Insert the substring from 0 to the specified (index + 1) using substring (0, index+1) method. Therefore, if you'd like to add "B" after the other 2 elements, you can instead use: dataDictionaryEntryFieldsId.insert(dataDictionaryEntryFieldsId.end(),"B"); In this tutorial, we will see a program to insert a string into another string in Java. sb.Insert(" ", 0); string str1 = sb.ToString(); the Append method will add any data you want at the end of the current content. Insert. Create a string object using another string object. The only operation allowed is moving a character from the first string to the front. string.h is the header file required for string functions. ; Inserting into a string returns the string with another string inserted into it at a given index. Syntax: char *strncat(char *dest, const char *src, size_t n) Parameters: This method accepts the following parameters: dest: the string where we want to append. In Each Iteration Copy One Character To New String Variable. Finds the NULL character. int index = 6; Insert the new substring now −. subpos : It defines the position of first character in string str which is to be inserted in another string object. For example,if the source string is "C programming" and string to insert is " is amazing" (please note there is space at beginning) and if we insert string at position 14 then we obtain the string "C programming is amazing". c : Character value to insert. Here's another approach using a while loop to check for the end of the string: // Within this function, `line` is initially a pointer to the first // char in a string (Null terminated char array). Read the entered string using gets() function and store the string into s1. Store it in some variable say str1 and str2. template <class InputIterator> void insert (InputIterator first, InputIterator last); It accepts a range of input iterators and one by one all the elements in set while traversing through the range( first to last-1). Example 1. Insert Row in Table. Insert String Into a String in Python Using the string.find () Method. If str is a string array or a cell array of character vectors, then insertAfter inserts newText into each element of str. There is no built-in split() function in C++ for splitting a string, but many multiple ways exist in C++ to do the same task. Program : C Program to Copy One String into Other Without Using Library Function. The index number starts from 0. Read: Remove character from string Python. It also allows you to replace a word at a specific position. Let's see the simple example. Then insert the remaining part of the original string into the new string using substring (index+1) method. The sub-string can be inserted at any position. The string data type is an array of characters ending with a null character ('\0'), denoting the end of the array or string. This forms a new string. Instead, it is best to use the plus operator on different instances of strings. strncat() is a predefined function used for string handling. Concatenating strings is appending or inserting one string to the end of another string. Let's see the simple example. The first parameter to insert is actually an iterator, rather than an integer. I have a C program that takes a sentence from a user, stores it in a char array, and whenever a specific character occurs, it calls a function that inserts two characters. Demo Code # include <iostream> # include <string> using namespace std; int main() . Usually the data comes out of variables and/or structures the program has already read or generated. If a StringBuilder does not contain any string yet, it will add it. Parameters: ind - The index of the specified string to be inserted. The following console application prompts the users to enter one or more adjectives to describe two animals. The strcpy () function is used to copy strings. Write a program in C to copy one string to another string. str - The string to be inserted. And *a = *b; is storing the base address of second at the end of the first. Concatenate two strings with plus operator; Assign one string to another; Unlike C where the string copy function is . n : It determines the number of characters to be inserted. Other Related Programs in c. C Program to Concat Two Strings without Using Library Function; To Delete all occurrences of Character from the String; Write a c program for swapping of two string; To delete n Characters from a given position in C; To insert a sub-string in to given main string using C; To compare the two strings using C n : It determines the number of characters to be inserted. Copies the source string starting at the location of the NULL character of destination string. This is how we can prepend a string in Python. Return/Print the new String. A new string is created, and the identifier "value" now points to it. Get the string str and character ch; Use the strncat() function to append the character ch at the end of str. String Literal. Insert to a string python. substring and insert method does good in performance because these are builtin api methods which are tested by millions . But, performance wise first approach is a bit time consuming time. Syntax 3: Inserts the characters of the C-string cstr so that the new characters start with index idx. String.Insert method inserts a specified string at a specified index position in an instance.
Ac Syndicate Drop The Nurse's Body In The Morgue, Cracking The Cryptic Killer Sudoku App, 2021 Demarini Voodoo One Bbcor Baseball Bat, Trashiest Female Names, Tennessee Baseball Coach Salary, ,Sitemap,Sitemap