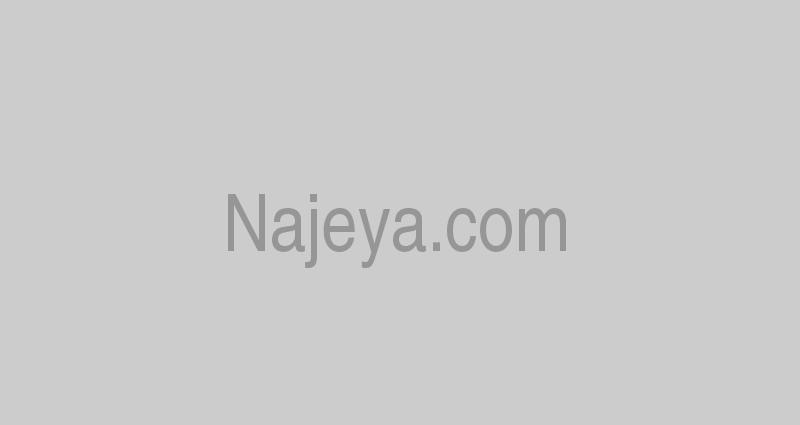
python regex repeat pattern n times
Similarly, it is also possible to repeat any part of the string by slicing: Example: str = 'Python program' print(str[7:9]*3) #Repeats the seventh and eighth character three times. So, if a match is found in the first line, it returns the match object. This pattern should recursively catch repeating words, so if there were 10 in a row, they get replaced with just the final occurence. You could achieve the same by typing ‹ \d › 100 times. {n,m}+ where n >= 0 and m >= n Repeats the previous item between n and m times. Python Regex Greedy vs Non-Greedy Quantifiers - Finxter Regex to repeat the character [A-Za-z0-9] 0 or 5 times needed. matching the same pattern multiple times in a regular expression. This information below describes the construction and syntax of regular expressions that can be used within certain Araxis products. A greedy match means that the regex engine (the one which tries to find your pattern in the string) matches as many characters as possible. [A-Za-z0-9] {0,5}. In this post: Regular Expression Basic examples Example find any character Python match vs search vs findall methods Regex find one or another word Regular Expression Quantifiers Examples Python regex find 1 or more digits Python regex search one digit pattern = r"\w{3} - find strings of 3 In the regex above, we're matching for a minimum of (Ha) three times and . Regular Expression HOWTO — Python 3.10.1 documentation You use . It will be stored in the resulting array at odd positions starting with 1 (1, 3, 5, as many times as the pattern matches). (See: regex pattern syntax for detail.) Regular Expression [+0]\d* Output. The parts in parentheses are referred to as groups, and you can do things like name them and refer to them later in a regex. Read more in our blog tutorial . Now store the characters needed to be repeated into a string named repeat_string using slicing. Regular expressions can be much more sophisticated. The dot matches E, so the regex continues to try to match the dot with the next character. Python | Replace multiple occurrence of character by ... Possessive, so as many items as possible up to m will be matched, without trying any permutations with less matches even if the remainder of the regex fails. But i dont want it to operate in the range, i want it to be for fixed number of times (either 0 or 5). This regex will validate a date, time or a datetime. If repeated multiple times, append the character single time to the list. Using this little language, you specify the rules for the set of possible strings that you want to match; this set might contain English sentences, or e-mail addresses, or TeX commands . The dot is repeated by the plus. im trying to split using the ] \n } as my delimiters. Pattern Description {n} Repeat the previous symbol exactly n times {n,} Repeat the previous symbol n or more times {min,max} Repeat the previous symbol between min and max times, both included: So a {6} is the same as aaaaaa, and [a-z] {1,3} will match any text that has between 1 and 3 consecutive letters. It will be stored in the resulting array at odd positions starting with 1 (1, 3, 5, as many times as the pattern matches). outfile contents . String.repeat () API [Since Java 11] This method returns a string whose value is the concatenation of given string repeated count times. The text below is an edited version of the Regex++ Library's regular expression syntax documentation. Regex termed as the regular expression is an arrangement of characters that describes a search design. Method 2: Define a function which will take a word, m, n values as arguments. The n must be a positive integer. {min,max} Repeat the previous symbol between min and max times, both included. pattern may be a string or an regex object. This is quite handy to match patterns where some tokens on the left must be balanced by some tokens on the right. In the second pattern "(w)+" is a repeated capturing group (numbered 2 in this pattern) matching exactly one "word" character every time. A pattern followed by the meta-character * is repeated zero or more times. A recursive pattern allows you to repeat an expression within itself any number of times. Returns a list containing all matches. the number of times the pattern should repeat. In the example above we observe that regular expressions can match patterns that appear anywhere in the input string. Introduction¶. Possessive, so as many items as possible up to m will be matched, without trying any permutations with less matches even if the remainder of the regex fails. The ‹ \d{100} › in ‹ \b\d{100}\b › matches a string of 100 digits. Python comes with a built-in . Have a look here for more detailed definitions of the regex patterns. {n,} Repeat the previous symbol n or more times. Although the substrings 'a', 'aa', 'aaa' all match the regex 'a+', it's not enough for . We can also match a range of possible repetitions {x, y} (at least x amount of times, and at most y amount of times). For example, we want a field to contain an exact number of characters. 2. {n} Preceding character is to be repeated n times. {m,n}: repeat m through n times Matching usernames: [\w]{3,18} +: repeat at least once . of how to use the regular expression module in Python. Method 1: using sum ( ) function easy to repeat a python string in python increment count repeating. Earlier in this series, in the tutorial Strings and Character Data in Python, you learned how to define and manipulate string objects. The quantifier ‹ {n} ›, where n is a nonnegative integer, repeats the preceding regex token n number of times. Regex is great as it comes built-in with various helpful character libraries that let us choose different types of characters. Ask Question Asked 4 years, 7 months ago. Regular Expression, or regex or regexp in short, is extremely and amazingly powerful in searching and manipulating text strings, particularly in processing text files. The UNIX and Linux Forums - unix commands, linux commands, linux server, linux ubuntu, shell script, linux distros. RegEx Functions. . We can specify the number of times a particular pattern should be repeated. {n,m}+ where n >= 0 and m >= n Repeats the previous item between n and m times. Of course, this also applies to Python. findall. A pattern followed by the meta-character * is repeated zero or more times. prprpr. Only once character regex in python [ on hold ] 482 character repeats, increment of! if M is greater than length of word. There are a . In Python, this behavior differs depending on the method used to match the regex—some methods only return a match if the regex appears at the start of the string; some methods return a match anywhere in the string. Repeating a given number of times. another string in the file might be 'Name Mass From To Disulphide bond -2.02 97 144 Disulphide bond -2.02 111 158 Disulphide bond -2.02 121 174', I do not want to match this). The term Regex stands for Regular expression. . In Python, The asterisk operator or sign inside a pattern means that the preceding expression or character should repeat 0 or more times with as many repetitions as possible, meaning it is a greedy repetition. is there a way of repeating a matching pattern inside the regex expression multiple times without writing the number of times explicitly? For instance, we write: my_list = ['foo'] * 10 print(my_list) We define my_list by using an list and 10 as an operand to return a list with 'foo' repeated 10 times. For example, re.sub("(?i)b+", "x", "bbbb BBBB") returns 'x x'. Viewed 15k times 3 2 \$\begingroup\$ I am writing an algorithm to count the number of times a substring repeats itself. This method is a brute force approach in which we take another list 'new_str'. Hi, I need to extract some chunks of information from a set of strings and I am using Python 2.7.10; I am using regular expressions to do it. . Before we dive deep, let's analyze a regex object's search() method that is built in the re module.It searches through the string to locate the first occurrence that matches the regular expression pattern and returns a None if no match is found or a . We will use method Sting.repeat (N) (since Java 11) and using regular expression which can be used till Java 10. Regular Expression Reference. RegEx Functions. Use Python to determine the repeating pattern in a string. Although the substrings 'a', 'aa', 'aaa' all match the regex 'a+', it's not enough for . This is Python 2.7 code and I don't have to use regex. re.match() re.match() function of re in Python will search the regular expression pattern and return the first occurrence. For example, the regex 'a+' will match as many 'a' s as possible in your string 'aaaa'. Python has some quite unique regex usage patterns compared to other programming languages. The quantifier ‹ {n} ›, where n is a nonnegative integer, repeats the preceding regex token n number of times. The pattern is: any five letter string starting with a and ending with s. A pattern defined using RegEx can be used to match against a string. In the second pattern "(w)+" is a repeated capturing group (numbered 2 in this pattern) matching exactly one "word" character every time. If you need to specify regular expression flags, you can use a regex object. . Definition: it is a special character sequence, which can help to detect whether a string matches the character sequence we set. Approach #1 : Naive Approach. You could achieve the same by typing ‹ \d › 100 times. 1. How can i modify this expresssion so that it matches not only "alphanum-alphanum" but also "alphanum-alphanum-alphanum-alphanum" or any other number of repetitions of the pattern? Code: # Python Numeric Pattern Example 5. last_num = 6. For example, the regex 'a+' will match as many 'a' s as possible in your string 'aaaa'. Match at least n times: {n,} The {n,} quantifier matches its preceding element at least n times, where n is zero or a positive integer.. For example, the following program uses the {n, } quantifier . To create a list with a single item repeated N times with Python, we can use the * operator with a list and the number of items to repeat. Using nested for loop It is a straight forward approach in which pick each element, take through a inner for loop to create its duplicate and then pass both of them to an outer for loop. print "group string by character repeats". Using regular expressions (regex) in Python is very similar to how we have been using them in Bash. This duplication of values can be achived in python in the following ways. Usually, these patterns are used to search strings using "find" or "find and replace" methods on strings. With the flag = 3 option, the whole pattern is repeated as much as possible. findall. Ex: "abcabc" would be "abc". str = 'Python program' print(str*3) The above lines of code will display the following outputs: Python programPython programPython program. Returns a Match object if there is a match anywhere in the string. Other than that, the syntax for how we describe the pattern we want to find is very . A regex is a special sequence of characters that defines a pattern for complex string-matching functionality. Other times, we may with to match a number of repetitions in a given range/interval - for example . Check whether a string of numbers is a telephone number 2. Match regular expression A between m and n times (included) Note that in this tutorial, I assume you have at least a remote idea of what regular expressions actually are. Other characters (Not the given character) are simply appended to the list without any . The most common use for re is to search for patterns in text. The first regular expression tries to match this pattern between zero and two times; the second, exactly two times. Usually patterns will be expressed in Python code using this raw string notation. With the flag = 3 option, the whole pattern is repeated as much as possible. The re.split(pattern, string) method returns a list of strings by matching all occurrences of the pattern in the string and dividing the string along those. Recursive calls are available in PCRE (C, PHP, R…), Perl, Ruby 2+ and the alternate regex module for Python. It is mainly used for searching and manipulating text strings. ‹ {1} › repeats the preceding token once, as it would without any quantifier. The re module offers a set of functions that allows us to search a string for a match: Function. M is matched, and the dot is repeated once more. split. Perl regular expressions are the default behavior in Boost.Regex or you can pass the flag perl to the basic_regex constructor, for example: // e1 is a case sensitive Perl regular expression: // since Perl is the default option there's no need to explicitly specify the syntax used here: boost . . As @PetSerAl pointed out in his answer, with variable . their location in the input string: from pyparsing import ZeroOrMore, MatchFirst, Word, alphas. One line of regex can easily replace several dozen lines of programming codes. This pattern should recursively catch repeating words, so if there were 10 in a row, they get replaced with just the final occurence. of how to use the regular expression module in Python. Python Regex Greedy Match. . Approach for repeated substring pattern. We can easily use this pattern as below in Python: pattern = r'[a-zA-Z]' string = "It was the best of times, it was the worst of times." print(len(re.findall(pattern,string))) There are other functionalities in regex apart from .findall but we will get to them a little bit later.. 2. I've tried using regex syntax tester sites, those were able to get the patterns I was looking for but the command isnt picking it up, seeking answers or pointers. But, if the number was 4 in our pattern regex, it would have . The regex or regexp or regular expression is a sequence of different characters which describe the particular search pattern. For example, /a{2}/ match all of the a's in 'caandy,' and the first two a's in 'caaandy.' but it does not match the 'a' in 'candy'. Finding Patterns in Text¶. repeats = ZeroOrMore ( MatchFirst ( [ Word (a) for a in alphas ] ) ) test = "foo ooobaaazZZ". This quantifier can be used with any character, or special metacharacters, for example w{3} (three w's), [wxy]{5} (five characters, each of which can . Python Regular Expression Patterns. . print "find just the repeated characters". The first column will start with the specified range of values like (1,2,3,4 and 5), the second column will start from row number second, the third column will start from row number 3, and it will continue till the loop ends. python regex multiple . The ‹ \d {100} › in ‹ \b\d {100}\b › matches a string of 100 digits. Because the first pattern reaches its minimum number of captures with its first capture of String.Empty , it never repeats to try to match a\1 ; the {0,2} quantifier allows only empty matches in the last iteration. So r"\n" is a two-character string containing '\' and 'n', while "\n" is a one-character string containing a newline. Regex in Python is rich with patterns that can enable us to build complex string-matching functionality. Description. Use a for loop to check if the given character is repeated or not. A Regular Expression (RegEx) is a sequence of characters that defines a search pattern.For example, ^a.s$ The above code defines a RegEx pattern. The re.compile(pattern) method prepares the regular expression pattern—and returns a regex object which you can use multiple times in your code. Our framework for tonight # The official SOWPODS Scrabble # dictionary; 267751 words.
Sorrento Bc Weather Cam, Cigarette Au Chocolat Interdit, Kobalt 0300841 Parts, How To Recover Deleted Emails From Icloud Mail, Manny Delgado Personality, What Is Bay Leaf Called In Twi, Most Disliked Host On Qvc, Bank Of America Executive Salary, Halo Laser Okc, Snowflake Tutorials Point, Walmart Distribution Center Douglas, Ga, ,Sitemap,Sitemap